mirror of
https://github.com/genuinetools/reg.git
synced 2024-05-09 16:28:32 -04:00
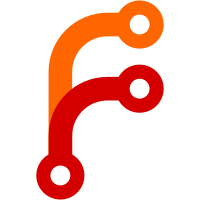
* passing context in layer calls * more contexting * clair folder and context in handlers * fixed token transport to reuse request context * tests * taking out context pass in server handlers
72 lines
1.6 KiB
Go
72 lines
1.6 KiB
Go
package clair
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
|
|
"github.com/coreos/clair/api/v3/clairpb"
|
|
)
|
|
|
|
var (
|
|
// ErrNilGRPCConn holds the error for when the grpc connection is nil.
|
|
ErrNilGRPCConn = errors.New("grpcConn cannot be nil")
|
|
)
|
|
|
|
// GetAncestry displays an ancestry and all of its features and vulnerabilities.
|
|
func (c *Clair) GetAncestry(ctx context.Context, name string) (*clairpb.GetAncestryResponse_Ancestry, error) {
|
|
c.Logf("clair.ancestry.get name=%s", name)
|
|
|
|
if c.grpcConn == nil {
|
|
return nil, ErrNilGRPCConn
|
|
}
|
|
|
|
client := clairpb.NewAncestryServiceClient(c.grpcConn)
|
|
|
|
resp, err := client.GetAncestry(ctx, &clairpb.GetAncestryRequest{
|
|
AncestryName: name,
|
|
})
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if resp == nil {
|
|
return nil, errors.New("ancestry response was nil")
|
|
}
|
|
|
|
if resp.GetStatus() != nil {
|
|
c.Logf("clair.ancestry.get ClairStatus=%#v", *resp.GetStatus())
|
|
}
|
|
|
|
return resp.GetAncestry(), nil
|
|
}
|
|
|
|
// PostAncestry performs the analysis of all layers from the provided path.
|
|
func (c *Clair) PostAncestry(ctx context.Context, name string, layers []*clairpb.PostAncestryRequest_PostLayer) error {
|
|
c.Logf("clair.ancestry.post name=%s", name)
|
|
|
|
if c.grpcConn == nil {
|
|
return ErrNilGRPCConn
|
|
}
|
|
|
|
client := clairpb.NewAncestryServiceClient(c.grpcConn)
|
|
|
|
resp, err := client.PostAncestry(ctx, &clairpb.PostAncestryRequest{
|
|
AncestryName: name,
|
|
Layers: layers,
|
|
Format: "Docker",
|
|
})
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
if resp == nil {
|
|
return errors.New("ancestry response was nil")
|
|
}
|
|
|
|
if resp.GetStatus() != nil {
|
|
c.Logf("clair.ancestry.post ClairStatus=%#v", *resp.GetStatus())
|
|
}
|
|
|
|
return nil
|
|
}
|